Ithaca High School Math Seminar Lesson 2-5
Date: 2023.11.15
The images we've made so far are static, i.e. they do not change with time. Today we'll start making dynamic animations.
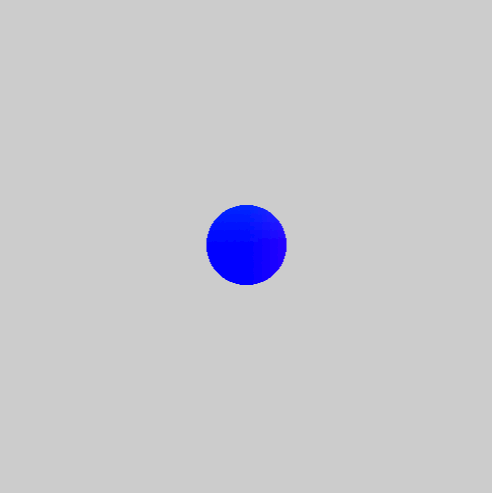
Step 1: Parametrize the radius
Let's begin by using something we basically made in the previous lesson.
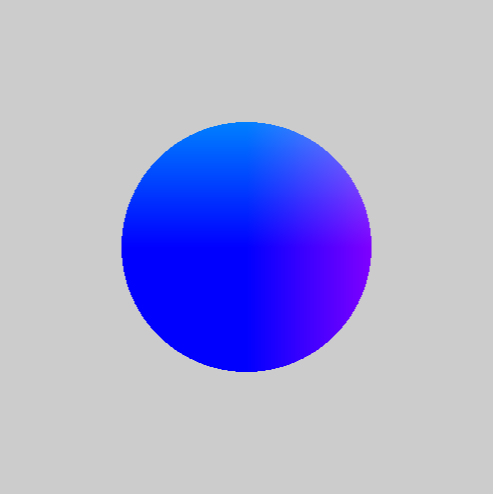
The image above is produced by the following shader code.
#version 330 uniform vec2 resolution; void main(void) { float screen_ratio = resolution.y / resolution.x; float xc = 2 * gl_FragCoord.x / resolution.x - 1; xc /= screen_ratio; float yc = 2 * gl_FragCoord.y / resolution.y - 1; vec4 color1 = vec4(xc,yc,1,1); vec4 color2 = vec4(0.8,0.8,0.8,1); bool in_R = xc * xc + yc * yc <= 0.25; gl_FragColor = float(in_R) * color1 + float(! in_R) * color2; }
The 0.25
here controls the size of the circle. We can make this value adjustable with a slider. First, in the right sidebar, click on the Project
tab, and scroll down until you find the Parameters
bar. Click the +
icon (pictured below). This will open a drop-down menu; choose Constant
→ Float
→ Float
.
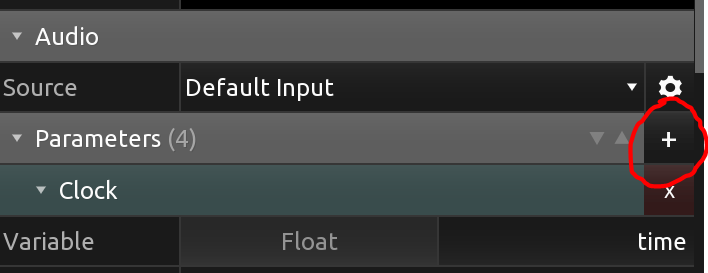
Scroll down to the bottom of the right sidebar; there should be options for a variable titled value1
(illustrated below). Change the name of value1
to radius
by clicking on the text value1
. Adjust the range of this variable by clicking on the bottom-right square with the filled-in dot (the bottom-right icon in the image below); set the range from to 0 to 1.
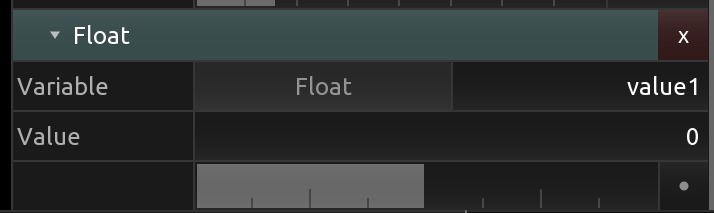
To use this variable, we should declare it as a uniform. Below or after the line uniform vec2 resolution;
add in:
uniform float radius;
Now replace the 0.25
in the code with radius
, and then slide the gray / dark gray bar in the radius options. The disk should change in size!
Step 2: Use the built-in uniform time
The last step was a fairly general method of defining and setting variables in fragment shaders we want to manually adjust. Kodelife comes with some built-in uniforms, one of which is time.
To use it, we only need change the line that we added from
uniform float radius;to
uniform float time;
We can now use this variable to create a radius which varies. For example, taking sin(time) would return a value between -1 and 1. Before the line in the fragment shader code where the bool in_R
is declared and set, then, we could add in the line
float radius = 1 + sin(time);
which would have the affect of periodically varying the radius from 0 to 2.
Task: Modify the code so that the radius of the disk varies over time.
A creative and often satisfying experience is making up your own animations.
Final Task: On your own or in a group, make a short and simple animation. (E.g. A disk which moves in a circle; a triangle which rotates over time.)