Ithaca High School Math Seminar Lesson 2-7
Date: 2023.11.21
This morning we'll look at the floor function, which can be used to create a variety of grid-based patterns. In particular, we'll create the checkerboard pattern below.
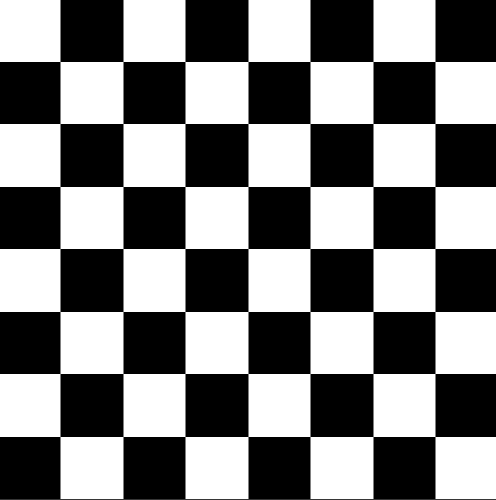
Step 1: Create by hand some graphs of variations of floor(x)
Define a function floor(x) that takes as input a real number and outputs a real number by the following rule: given a real number a, floor(a) is the smallest integer that is less than or equal to a.
Task: Create a plot of floor(x) by on paper (or the digital equivalent) on the interval [-2,2]. Now do the same for floor(2x) and floor(x/2). Repeat for 2 × floor(x) and 0.5 × floor(x). Finally, graph 2 × floor(2x) + 2 on [-2,2].
Step 2: Create a checkerboard pattern
Let's adapt the code from lesson 2-5: open up a new file in KodeLife, delete all the default code, and paste the code which draws a blue disk.
#version 330 uniform vec2 resolution; void main(void) { float screen_ratio = resolution.y / resolution.x; float xc = 2 * gl_FragCoord.x / resolution.x - 1; xc /= screen_ratio; float yc = 2 * gl_FragCoord.y / resolution.y - 1; vec4 color1 = vec4(xc,yc,1,1); vec4 color2 = vec4(0.8,0.8,0.8,1); bool in_R = xc * xc + yc * yc <= 0.25; gl_FragColor = float(in_R) * color1 + float(! in_R) * color2; }
If we replace the line which sets in_R
to the line
bool in_R = int(floor(xc)) % 2 == 0;
and adjust color1
and color2
to be black and white and make the aspect ratio a square, we get the following image.
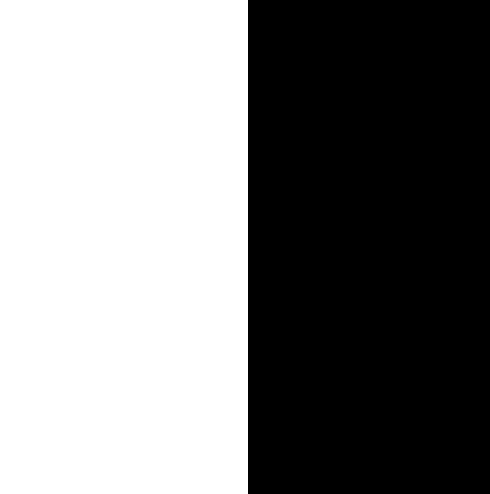
Here wrapping floor(xc)
in int()
converts the data type of the result of floor(x)
from a float to an integer, and then the % 2
takes the remainder of this integer when divided by 2. There is another way to do this calculation using bitwise operators, but more on this in a later lesson.
Task: Adjust the same line above so that the image below is obtained. Hint: consider the variations of floor(x) you plotted in Part 1.
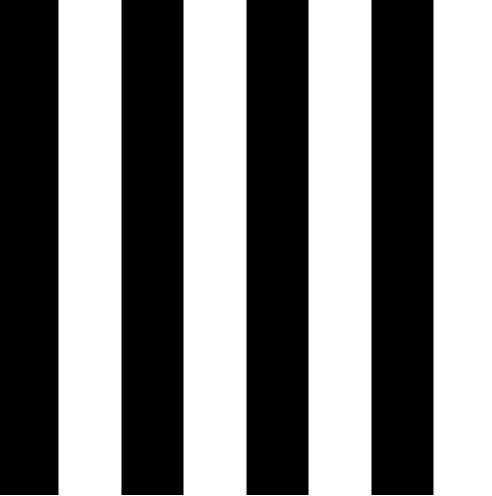
We are now ready to make the image at the top of this page.
Final task: Adjusting the same line or otherwise, create a checkerboard pattern.